We've created a separate section for each app in the Let's App! booklet. Here they are:
Dancin' Hal
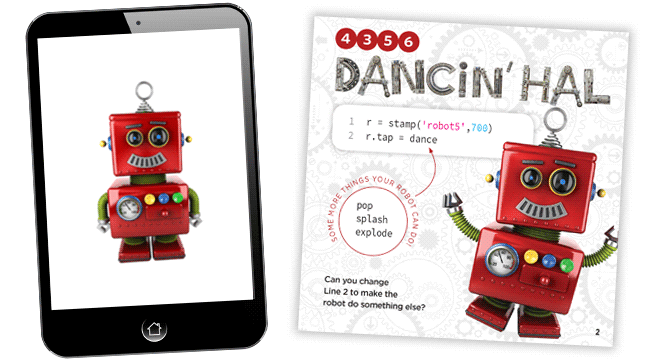
This simple app puts a friendly robot on the screen. When kids tap it, it dances.
Line 1 stamps a robot on the screen and assigns it the name "r".
- 'robot5' is the label of the robot graphic we want the app to use.
- 700 is the size of the robot on the screen, in pixels.
Line 2 tells "r" (which is the robot) to dance when it's tapped.
- The dance function is built into Bitsbox.
- pop, splash and explode are other built-in functions you can use here.
So Long, Homework!
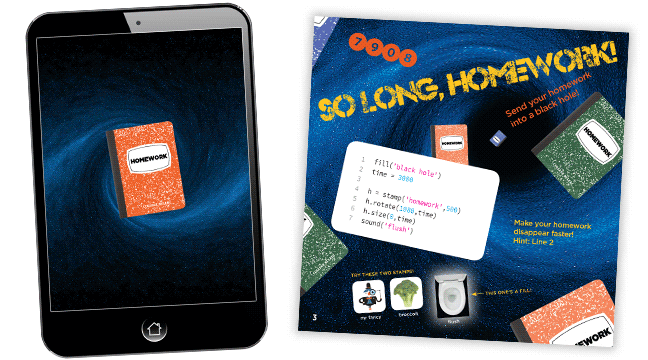
This app puts a picture of a homework book on the screen. Without doing anything, the book spins and shrinks until it disappears into a black hole background image. A flushing sound effect plays while the book is disappearing.
Line 1 puts a background image of a black hole on the screen.
- 'black hole' is the label of the graphic we want the app to use.
Line 2 creates a new variable called "time" and assigns it the value 3000.
- This variable will be used on lines 5 and 6.
Line 3 is left blank to create visual spacing in the code.
Line 4 stamps a homework book on the screen and assigns it the name "h".
- 'homework' is the label of the book graphic we want the app to use.
- 500 is the size of the book on the screen, in pixels.
Line 5 tells "h" (which is the book) to rotate.
- 1080 is the number of degrees it should rotate, which equals 3 full rotations.
- The value of time is 3000, so the book rotates for 3000 milliseconds (3 seconds).
Line 6 tells "h" (which is the book) to change size.
- 0 is the size (in pixels) it should end up when it's finished.
- The value of time is 3000, so the book shrinks to 0 in 3000 milliseconds (3 seconds).
Line 7 plays a sound.
- 'flush' is the label of the sound file we want the app to use.
BlockCraft
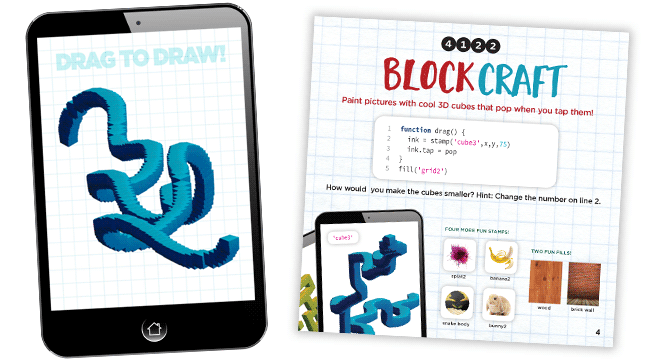
This app lets you draw on the screen to make cool 3D effects. Dragging on the screen draws with hundreds of little cubes. Tapping a cube makes it pop and disappear.
Line 1 creates a new block of code called drag() which tells the app what to do when you drag your cursor on the screen.
- The drag() function is built into Bitsbox.
- A function is a block of code which only runs when it's called. In this case, the drag() function is called whenever someone drags on the screen.
Line 2 puts a blue cube on the screen and assigns it the name "ink". The cube appears precisely where your cursor is touching the screen.
- 'cube3' is the label of the cube graphic we want the app to use.
- x and y refer to where you're touching on the screen.
- 75 is the size of the cube on the screen, in pixels.
Line 3 tells "ink" (which is the cube) to pop when it's tapped.
- The pop function is built into Bitsbox.
Line 4 uses a curly bracket } to indicate that this is the end of the drag() function which started on line 1.
Line 5 puts a background image of graph paper on the screen.
- 'grid2' is the label of the graphic we want the app to use.
Dig, Bunny, Dig!
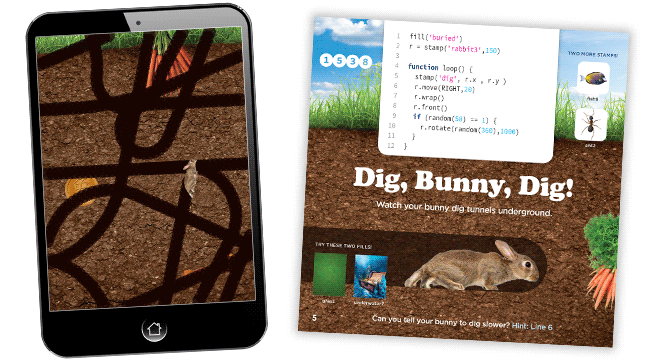
This app sends a rabbit burrowing all over the screen. Her movements are random, and the pattern she draws with her tunnels is different every time.
Line 1 puts a background image of an underground world on the screen.
- 'buried' is the label of the graphic we want the app to use.
Line 2 stamps a rabbit on the screen and assigns it the name "r".
- 'rabbit3' is the label of the bunny graphic we want the app to use.
- 150 is the size of the bunny on the screen, in pixels.
Line 3 is left blank to create visual spacing in the code.
Line 4 creates a new block of code called loop() which tells the app to repeat everything inside it 20 times per second, forever.
- The loop() function is built into Bitsbox.
- A function is a block of code which only runs when it's called. In this case, the loop() function begins automatically as soon as the app starts running.
Line 5 stamps a black circle at the same screen location as the rabbit. Put together, hundreds of black circles combine to look like a tunnel.
- 'dig' is the label of the graphic we want the app to use.
- r.x and r.y refer to the current location of "r" (the rabbit) on the screen.
Line 6 tells "r" (which is the rabbit) to move.
- RIGHT is the direction the rabbit should move.
- 20 is the distance, in pixels, that the rabbit should move.
Line 7 tells "r" (which is the rabbit) to "wrap" around if it gets to the edge of the screen. If it goes off the top, it appears at the bottom, and so on.
Line 8 tells "r" (which is the rabbit) to appear on top of the stamp 'dig'.
- Without this line of code, the rabbit would be hidden behind the tunnel.
Line 9 begins an if statement. If the expression between the parentheses is true, the code on line 10 should run. If the expression is false, the code doesn't run at all.
- This line of code picks a random number from 1 to 50 and checks to see if it's equal to 1.
- The command random(50) generates a random number from 1 to 50.
- The operator == means "is equal to".
- The point of this line of code is to make the rabbit turn about once every 50 steps. Sometimes it's more frequently and sometime it's less.
Line 10 tells "r" (which is the rabbit) to rotate by a random amount, and to take 1 second to do it.
- The command random(360) generates a random number between 1 and 360.
- The result of the command random(360) is the number of degrees it should rotate.
- 1000 milliseconds (1 second) is the length of time it should take to rotate.
Line 11 uses a curly bracket } to indicate that this is the end of the if statement which started on line 9.
Line 12 uses a curly bracket } to indicate that this is the end of the loop() function which started on line 4.
Red Racer
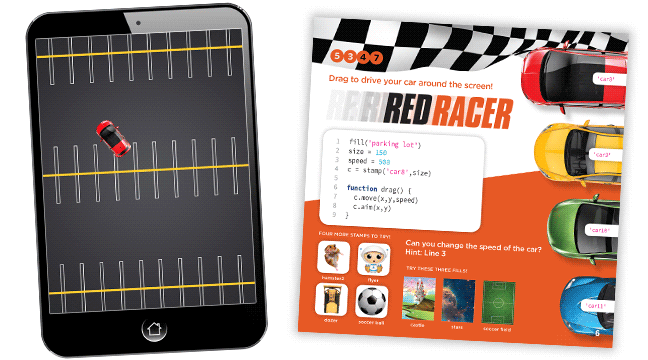
This app lets you drive a car by dragging your cursor on the screen.
Line 1 puts a background image of a parking lot on the screen.
- 'parking lot' is the label of the graphic we want the app to use.
Line 2 creates a new variable called "size" and assigns it the value 150.
- This variable will be used on line 4.
- This variable exists to make this app easier to modify.
Line 3 creates a new variable called "speed" and assigns it the value 500.
- This variable will be used on line 7.
- This variable exists to make this app easier to modify.
Line 4 stamps a car on the screen and assigns it the name "c".
- 'car8' is the label of the car graphic we want the app to use.
- The value of size is 150, so the size of the car is 150 pixels.
Line 5 is left blank to create visual spacing in the code.
Line 6 creates a new block of code called drag() which tells the app what to do when you drag your cursor on the screen.
- The drag() function is built into Bitsbox.
- A function is a block of code which only runs when it's called. In this case, the drag() function is called whenever someone drags on the screen.
Line 7 tells "c" (which is the car) to move.
- x and y refer to where you're touching the screen.
- The value of speed is 500, so the car takes 500 milliseconds (0.5 seconds) to move.
Line 8 tells "c" (which is the car) to rotate until it's aimed at the spot where you're touching the screen.
- x and y refer to where you're touching the screen.
Line 9 uses a curly bracket } to indicate that this is the end of the drag() function which started on line 6.
Click on an app name above to view the code translated content!